-
Arrays for Python
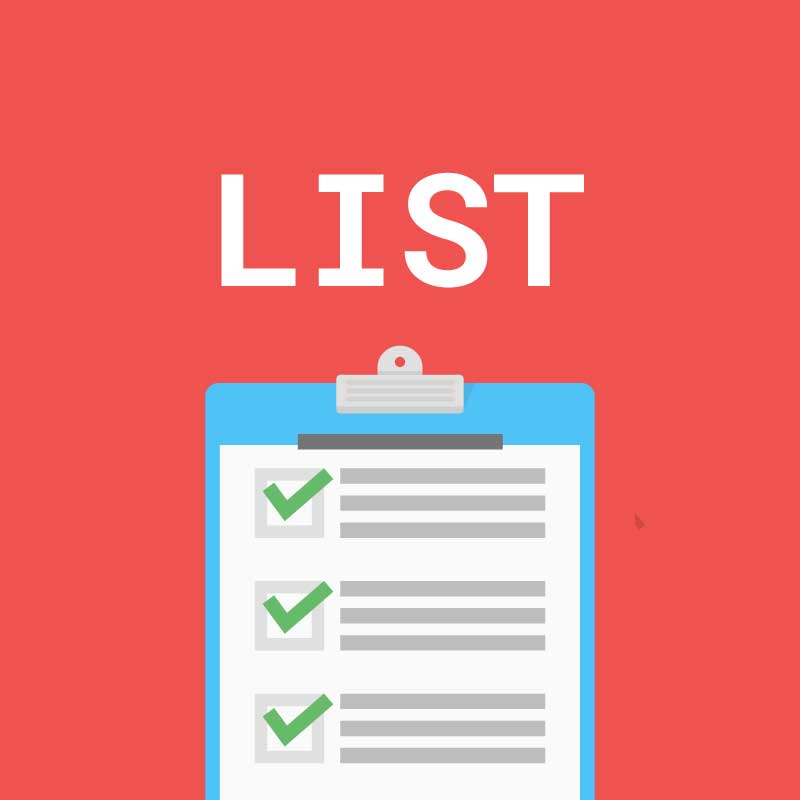
We are continuing our series of articles about the data structures in Python. In the previous one we've been talking about the Python dictionaries, and here we'll cover the topic of Array Data Structures.
A fundamental data structure in any programming language that's widely used across different algorithms is called the array. Simply put an array is a group of related elements sharing a common name. Arrays are considered to be the contiguous data structures because they store information in the adjacent memory blocks. They hold fixed size data records and each element can be fast and successfully located by its index.
You can find several array-like ones in its library. That's why here we'll take a quick look on a few of them: list
, tuple
, array.array
, and of course another one worth mentioning is NumPy that provides a great array object, because it has a built in function for creating arrays. But we'll get back to this one later.
Lists
So, the list in Python is an ordered collection of items that can be accessed by iterating over the list. And those items can be of any type which might result in more space taken up if supporting multiple data types. Lists are a part of the core Python language and they allow to add and remove elements after what the memory will be automatically allocated or released. This is due to Python list being a dynamic mutable type. In the previous article on the common beginner mistakes in Python we've been talking about the frequent misactions of copying lists. Lists are enclosed in square brackets:
>>> elements = ['nick', 'john', 'alex'] >>> elements[1] 'john' >>> elements[2] = 'alisa' >>> elements ['nick', 'john', 'alisa'] >>> elements.append(17) >>> elements ['nick', 'john', 'alisa', 17]
Here is a trick available for Python 3 only:
>>> a, *b, c = [1, 2, 3, 4, 5] >>> a 1 >>> b [2, 3, 4] >>> c 5
Tuples
Tuples are similar to lists in a way that they can contain any type of objects, which makes them flexible, and they are also the part of the standard Python language. But they also differ, because Python tuples are immutable which means that it's no longer possible to add and remove elements dynamically. And due to the fact the tuples don't have the append method (like lists) you have to create a new copy of a specific tuple with an extra element. Tuple elements can be accessed through the unpacking or indexing. They have to be defined first up and you won't be able to change their values. The size of a tuple makes it faster than the list.
a = tuple(range(1000)) b = list(range(1000)) a.__sizeof__() # 8024 b.__sizeof__() # 9088
But it's still not enough for them to occupy less memory than a typed array. Tuples are enclosed in parentheses:
>>> elements = ('nick', 'john', 'alex') >>> elements[0] 'nick' >>> elements ('nick', 'john', 'alex') >>> elements[1] = 'alisa' TypeError: "'tuple' object does not support item assignment" >>> del elements[1] TypeError: "'tuple' object doesn't support item deletion" >>> elements + (17,) ('nick', 'john', 'alex', 17)
Tuples can be used as dictionary keys or set elements due to them being immutable, and that's the requirement that lists don't meet.
>>> dd = { ('John', 'Snow'): 'Stark', ('Robert', 'Baratheon'): 'Big guy' } >>> dd[('John', 'Snow')] 'Stark' >>> dd = { ['John', 'Snow']: 'Stark', ['Robert', 'Baratheon']: 'Big guy' } TypeError: unhashable type: 'list'
array.array
An efficient array storage type is implemented by the array module. It's different from the lists and tuples in a way that all the elements have to be of the same type - the basic C-style data type (like bytes), which is defined during the array creation. And all of the Python arrays generated with the array.array class are mutable. Array.array objects occupy less space and give the opportunity to store many elements as long as they have the same type. There are several methods to array object and many of them are the same as regular lists methods, so you can use them.
>>> import array >>> elements= array.array('f', (2.3, 2.6, 2.9, 3.2)) >>> elements[1] 2.6 >>> elements array('f', [2.3, 2.6, 2.9, 3.2]) >>> elements[1] = 35.6 >>> elements array('f', [2.3, 35.6, 2.9, 3.2]) >>> del elements[1] >>> elements array('f', [2.3, 2.9, 3.2]) >>> elements.append(51.0) >>> elements array('f', [2.3, 2.9, 3.2, 51.0]) >>> elements[1] = 'nick' TypeError: "must be a real number, not str"
numpy.array
As it has been mentioned above the great alternative to Python array-like data structures is NumPy (Numerical Python) which can be easily installed. It's a fundamental array processing package for scientific computing in the Python programming language that was created for the efficient manipulation of the large multidimensional arrays due to its hard-core N-dimensional array object ndarray. In comparison to other builtin Python data structures it's much more efficient for storing data. It serves as the ultimate container for data to be passed between algorithms. NumPy can create arrays of arbitrary type, read and write array-based data sets to disk and has many high-level mathematical functions which allows it to make componentwise computations with arrays and operations between them. It also contains tools for integrating C/C++ and Fortran code and can perform random number generation, linear algebra operations, and Fourier transform. NumPy can without delay and difficulty integrate a wide variety of databases. It's available for Python 2 and 3.
An object that wraps a static array of different data types and is completed with methods is a NumPy array. You can have two types of array-like entity within your program due to the fact that NumPy array is a completely separate data type. But it's easy to convert an array-like Python data types to NumPy arrays. The last differ from the Python ones in two aspects. The first one is that all of the NumPy arrays are of the same type, and the second one is that once generated a NumPy array size can't be changed.
Here's an example of working with NumPy.
>>> import numpy as np >>> arr = np.array([2, 4, 8]) >>> arr array([2, 4, 8]) >>> arr * 2 array([ 4, 8, 16]) >>> arr + np.array([3,2,1]) array([5, 6, 9])
Due to the fact that NumPy is designed for dealing with the large data sets it provides a wide range of functionalities for massive data modifications.
A low level constructor ndarray
can be used to create a NumPy array. But there are other ways, like:
np.zeros(shape) which will create an array of the specified shape initialized to zeros.
>>> np.zeros((2, 3)) array([[ 0., 0., 0.], [ 0., 0., 0.]]
Similarly np.ones(shape) method will create an array filled with 1 values.
np.arange will create a one dimensional array with regularly incrementing values.
>>> np.arange(10) array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]) >>> np.arange(2, 10, dtype=np.float) array([ 2., 3., 4., 5., 6., 7., 8., 9.]) >>> np.arange(2, 3, 0.1) array([ 2. , 2.1, 2.2, 2.3, 2.4, 2.5, 2.6, 2.7, 2.8, 2.9])
The np.linspace method will generate an array of specified size with equally spaced values.
>>> np.linspace(1., 4., 6) array([ 1. , 1.6, 2.2, 2.8, 3.4, 4. ])
And np.indices will create a set of arrays (one array per dimension) where each array represent a variation in that dimension.
>>> np.indices((3,3)) array([[[0, 0, 0], [1, 1, 1], [2, 2, 2]], [[0, 1, 2], [0, 1, 2], [0, 1, 2]]])
The array method can be used to convert a Python array object into a NumPy array.
myArray=np.array([[1,2,3],[4,5,6],[7,8,9]])
Conclusion
So, here we've reviewed four arrays in Python. If this article was useful and you'd like to found out more on the Python data structures, then wait and check out our next article about class generators. Feedbacks are highly welcomed.
P.S.
Here are the missions that might be interesting to solve in order to practice using arrays: Non-unique Elements where you have to return a list with non-unique elements by removing the unique once, and Most Numbers in which you need to determinate the difference between the maximum and the minimum elements of the assigned array of numbers.
Also you can find examples of array manipulation in the solution for ADFGVX Cipher mission by Sim0000.
Welcome to CheckiO - games for coders where you can improve your codings skills.
The main idea behind these games is to give you the opportunity to learn by exchanging experience with the rest of the community. Every day we are trying to find interesting solutions for you to help you become a better coder.
Join the Game