-
New Python on CheckiO
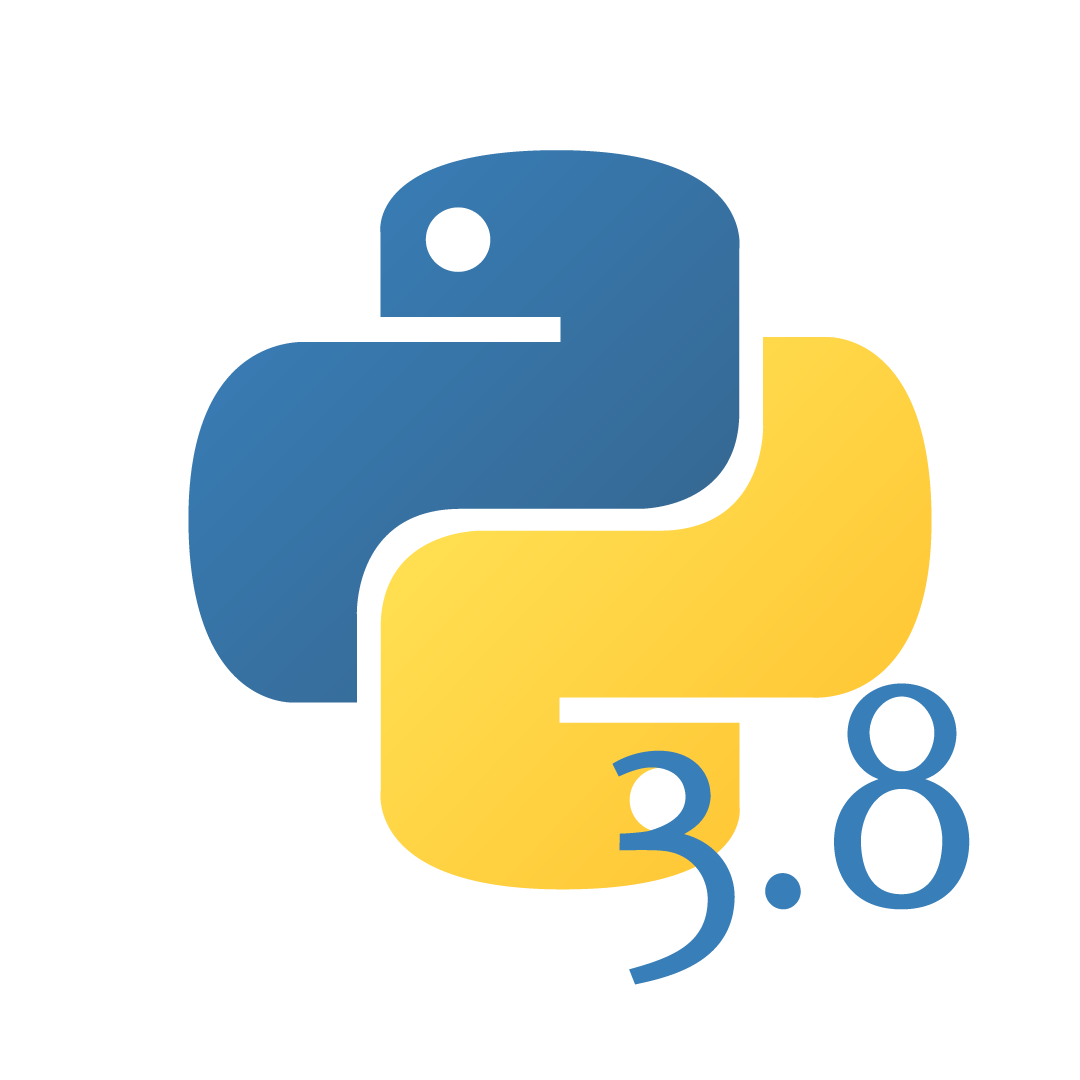
Hi, guys!
There are some cool changes that I’m excited to share with you. We’ve updated the Python version that has been used on CheckiO to the latest Python 3.8 and, while it’s in alpha testing, all CheckiO users are able to try it out right now.
Here I wanted to highlight some of the newly available features.
The first one is the assignment expressions.
This feature allows you to assign values to variables within an expression. The syntax of a named expression has a form of NAME := expr
. It’s highly useful to name the result of an expression, since it gives you the opportunity to use a descriptive name instead of a longer expression and reuse it if needed. Also it can favor an interactive debugger when you name sub-parts of a large expression.
Example:
if (n := len(a)) > 10: print(f"List is too long ({n} elements, expected <= 10)")
The assignment expressions don’t support multiple targets, assignments not to a single name, iterable packing/unpacking, inline type annotations, and augmented assignment. They are better used for simplifying list comprehension and getting conditional values. Also assignment expressions maintain the current scope and honors if the scope contains a nonlocal or global declaration for the target. But there is also TargetScopeError added for the invalid cases.
The := operator (or the “walrus operator”) can improve code readability and make it more compact. It should always have spaces around it and is never allowed at the same level as =. It can be used directly in a positional function call argument and in contexts where statements are illegal.
You can read more about it in PEP 572.
The second feature is the positional-only parameters.
As we know arguments can be passed to Python functions by position or by keyword. But there are cases when it’d be more preferable to restrict some function parameters to only be passed by position. Well, the positional-only parameters can be quite useful in those cases. But first, what do they actually do? They make possible for the function parameters to be specified positionaly, so based on their order. This introduced a new syntax / .
Example:
def pow(x, y, z=None, /): r = x**y if z is not None: r %= z return r
This feature provides library authors with the control over the way their API would be called and opportunity to better express the intended usage of an API. By specify positional-only parameters the name of the parameters can be easily changed.
There are also performance benefits due to the fact that parsing and handling of positional-only arguments is faster.
Besides that, they make it easier to maintain pure Python implementations of C modules and can exert some logical order when calling interfaces where they are applied.
So, the most obvious situations when positional-only parameters can be of use are: when a function can accept both keyword and positional arguments (Formatter.format
and dict.update
); when there is no external semantic meaning for the parameter name; when an API's parameters are required and precise.
Positional-only parameters can make Python language more consistent and also allow some new optimizations, which you can find out more about them along with some other things in PEP 570.
And the last feature that I’ll cover here is the f-strings now support = for quick and easy debugging.
f-strings are uniquely able to help make the debugging process much easier and less time consuming. They compile the original text of the expression which they require during implementation.
By adding = specifier to f-string you can expand the text of the expression f'{expr=}'
and see the repr of the evaluated expression.
Example:
x = 3 print(f'{x*9 + 15=}')
The outcome would be x*9 + 15=42
.
It’s also composable with conversions and you can use whitespaces around the equal sign no problem.
Check out bpo-36817 for more info.And it's just the tip of the iceberg! We are eager to hear your thoughts in the comments below and you can already start trying those new features of Python 3.8 out by solving the following new missions:
“Sum Consecutives” where you need to sum strictly the identical and consecutive numbers in a given list of integers.
“Split Pairs” where your task is to split the string into pairs of two characters and replace the missing one if the string contains an odd number.
Welcome to CheckiO - games for coders where you can improve your codings skills.
The main idea behind these games is to give you the opportunity to learn by exchanging experience with the rest of the community. Every day we are trying to find interesting solutions for you to help you become a better coder.
Join the Game