-
Cryptography for dummies: encryption with the examples
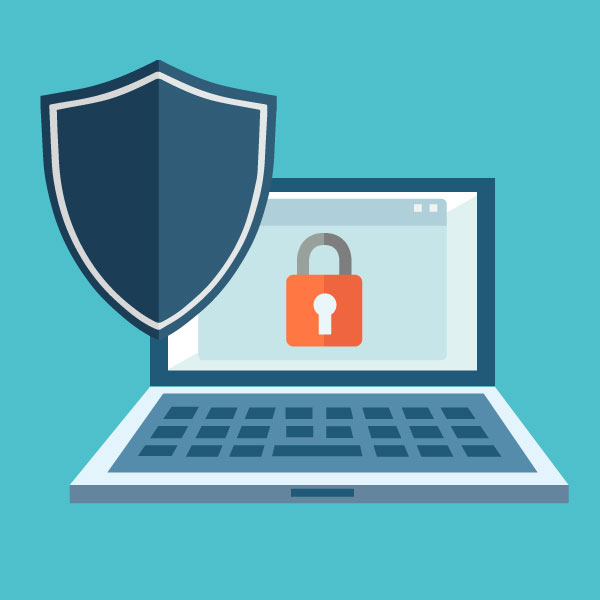
Every person who uses a computer or a smartphone also faces cryptography every day: starting from working on the Internet over HTTPS protocol and ending with the notorious software viruses. However, not many understand how cryptography actually works. Let’s try to sort it all out on specific examples.
Wikipedia says: Cryptography (from Greek κρυπτός kryptós, "hidden, secret"; and γράφειν graphein, "writing") – is the study of techniques used to ensure confidentiality (an inability for the outsiders to read the information), data integrity (an impossibility to inconspicuously change the information), authentication (a verification of the authorship or other object properties) and non-repudiation.
Encryption is one specific element of cryptography. During the process of encryption with the help of a certain secret a reversible change of information occurs which makes it hidden for those who don’t know the secret. The basic principles of this process we’ll review in the article.
Symmetric encryption
Let's suppose that the participant A wants to send the participant B some secret information. Stop. A, B – it’s not cool or comfortable. That’s why in cryptography it is customary to call the information exchange participants by names Alice (the sender) and Bob (the intended recipient).
Now, how can Alice send the information so that nobody but Bob could read it? It’s necessary to somehow change the data according to an algorithm previously agreed with Bob. The easiest way to implement such a task is a substitution cipher, an algorithm in which each letter of a message is replaced by another letter. For example, instead of the first letter of the alphabet (“A”) Bob and Alice will use the third letter (“C”), instead of the second (“B”) – the fourth one (“D”), and so on.
In this case, the encryption algorithm is an alphabet shift, the letters are being shifted forward and number 2 is the key (shifted by two spaces). Everyone who knows the algorithm and the key can decipher Alice’s message. A simple example in Python 3:
ALPHA = u'abcdefghijklmnopqrstuvwxyz' def encode(text, step): return text.translate( str.maketrans(ALPHA, ALPHA[step:] + ALPHA[:step])) def decode(text, step): return text.translate( str.maketrans(ALPHA[step:] + ALPHA[:step], ALPHA))
These kinds of encryption algorithms, in which Alice and Bob previously have to come up with and then agree on the same secret, are called symmetric-key algorithms. And the example above is the simplest algorithm of this group called the Caesar cipher. It’s considered unsafe and not recommended for using. The most popular and cryptographically secure symmetric-key algorithms are 3DES and AES.
Asymmetric encryption
But what to do if Alice and Bob are too far away from one another and cannot agree on the use of the same shared secret, cause there is a certain Eve (“the eavesdropper” for the adversary) who wants to find out Alice and Bob’s secrets. In this case, Bob can send Alice a lock, the key to which only he has. Alice will put the letter into the box and secure it with that lock. Now neither Alice nor Eva will be able to open the box and read the letter.
A similar approach is used in asymmetric encryption, also known as public-key cryptography. In the example with Alice and Bob, Bob's secret key will be the key to the lock, and the public key we can call the lock itself. Sending Alice a lock is a key agreement algorithm.
The most popular public key encryption algorithm is RSA. Here's how its implementation looks like in Python using the RSA library:
import rsa #Bob forms a public and a secret key (bob_pub, bob_priv) = rsa.newkeys(512) #Alice forms a message to Bob and encodes it in UTF8, #because RSA operates only on bytes message = 'hello Bob!'.encode('utf8') #Alice encrypts the message with Bob's public key crypto = rsa.encrypt(message, bob_pub) #Bob decrypts the message with his secret key message = rsa.decrypt(crypto, bob_priv) print(message.decode('utf8'))
It should also be noted that asymmetric algorithms work slower than symmetric ones, and very often a scheme can be found when an asymmetric algorithm encrypts a symmetric secret and further exchange of messages occurs according to a symmetric algorithm.
Conclusion
In this article we reviewed only one of the cryptography elements – encryption. If this topic was interesting for you then you are welcome to follow our further publications and try out some of the missions on CheckiO: Cipher Map, Vigenere Cipher, The Hidden Word etc.
Welcome to CheckiO - games for coders where you can improve your codings skills.
The main idea behind these games is to give you the opportunity to learn by exchanging experience with the rest of the community. Every day we are trying to find interesting solutions for you to help you become a better coder.
Join the Game